Linux Basics
Learn the basics of operating a Linux-based operating system (OS) and take your first steps in exploitation in a Linux environment!
Easy
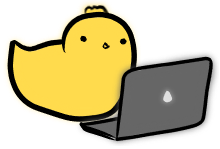
Linux_Basics::TCP_and_Pwntools
Networking is a necessity with modern technologies, and TCP (transmission control protocol) is an essential protocol that drives much of the network traffic in modern systems. We will go through a high-level overview of TCP.
Pwntools is a Python library that boasts some useful features for dealing with TCP connections. It allows you to automate interaction with executables as well. While this is not a Linux-specific tool, we believe it is very useful and knowing how to use it will be an essential skill for completing many of the challenges provided.
TCP
As mentioned earlier, TCP is short for transmission control protocol. TCP is a protocol or a set of rules and procedures to allow data packets to be reliably sent between computers. TCP is used on top of IP (internet protocol). While these terms may be confusing, the specifics on their inner workings are unimportant for most work we will do. However, as a general overview, the IP helps to get small data packets across connections, while TCP helps to make sense of these packets and allow them to translate into understandable messages for either party on a connection.
Where TCP is used
While you may or may not have heard of TCP, it is a ubiquitous standard and is used in many of the technologies that you use in every day life.
Here are some examples of other protocols that are built on top of TCP:
- SMTP (the protocol used to send and receive emails)
- HTTP/HTTPS (the protocol used by internet browsers to communicate with websites)
- SSH (the protocol used to remotely connect to servers)
IP addresses
An essential concept to understand when interacting with TCP services is the ip address.
An IP address is a unique address (a fancy way to represent a number) that identifies a specific device on a network like the internet. When you wish to communicate with another device on a network, it is important to be aware of their IP address so that you are able to send your data to the right device.
The current version of IP being used is IPV4, and has addresses that look like 4 dot-separated numbers. Here are some example IP addresses:
- 127.0.0.1
- 8.8.8.8
- 192.168.1.1
However, IP addresses are not easy to remember, and thus there is an additional protocol (DNS), which allows people to associate domain names with their IP addresses. This way, people who wish to communicate with those devices, do not need to remember an IP, and can instead issue a DNS query to figure out the IP address, only knowing the domain name.
For example, if we browse to google.com, our browser will first make a DNS query to figure out the corresponding IP address for the google.com servers.
To simulate this process we can use the dig
command.
$ dig google.com
; <<>> DiG 9.16.1-Ubuntu <<>> google.com
;; global options: +cmd
;; Got answer:
;; ->>HEADER<<- opcode: QUERY, status: NOERROR, id: 43813
;; flags: qr rd ra; QUERY: 1, ANSWER: 1, AUTHORITY: 0, ADDITIONAL: 1
;; OPT PSEUDOSECTION:
; EDNS: version: 0, flags:; udp: 65494
;; QUESTION SECTION:
;google.com. IN A
;; ANSWER SECTION:
google.com. 5 IN A 172.217.162.110
;; Query time: 4 msec
;; SERVER: 127.0.0.53#53(127.0.0.53)
;; WHEN: Thu Jul 29 22:19:36 +08 2021
;; MSG SIZE rcvd: 55
Notice the ;; ANSWER SECTION
contains the IP address 172.217.162.110
.
That is the IP address that my internet browser will send data to and receive data from in order to show me the google.com web page.
In general, most tools that allow users to connect to other devices on the network will handle this process automatically, so it is usually not necessary to resolve the IP addresses ourselves. This demonstration is largely for explanation sake.
Quiz
Which of the following protocols is not used on top of TCP?
Ports
At this point, we should understand that IP addresses (generally) map to a single device on a network, and allow us to identify to communicate with. However, this is not the only information we require if we wish to communicate with the device through TCP.
If we think of IP addresses as physical addresses in the real world, TCP helps describe what information should be included in a parcel.
However, just like the world has apartment buildings that house multiple homes, a single IP address may have multiple TCP services. In the real world, the specific apartment number is then required in order to deliver a parcel to the right recipient. Similarly, IP packets sent over TCP should specify the port, which is the apartment number equivalent that specifies which services in a single server the packet is intended for.
In TCP, a port is just an integer from 1 to 65535. This way, a single server can run multiple services on separate ports. Any connections that need to communicate with these services can then specify the corresponding port to send data to the right service.
Quiz
Which of the following numbers cannot be used as a valid TCP port?
Netcat
So how can we make TCP connections manually ourselves?
A useful command for this is nc
(netcat).
The syntax for nc
is very simple:
nc <destination ip/domain> <port>
We can try this with google.com. The common port for HTTP, the protocol that runs the web, is 80 generally.
$ nc google.com 80
GET /
This will give us a large response from the server.
HTTP/1.0 200 OK
...
We have succesfully made a TCP connection!
If we wish to use the IP address instead, that will be valid as well.
$ nc 172.217.162.110 80
Pwntools
Now let's try to repeat the same steps using the Pwntools library instead! Make sure to have pwntools installed. We will be using Python3 in our lesson example, but most code should be similar even if you use Python2.
$ pip install pwntools
To make the same connection using Pwntools, our script will look like so.
#!/usr/bin/env python3
# Import all from pwntools library
from pwn import *
# Create connection with remote service
conn = remote("google.com", 80)
# Send data request
conn.send(b"GET /\r\n")
# Receive one line of reponse from server
line = conn.recvline()
print(line)
# Close connection when we are done
conn.close()
We can run this and see if it works!
$ chmod +x script.py
$ ./script.py
[+] Opening connection to google.com on port 80: Done
b'HTTP/1.0 200 OK\r\n'
[*] Closed connection to google.com port 80
We get the same HTTP/1.0...
first line response as earlier. Success!
This script just showcases a small subset of features Pwntools provides for automation. Pwntools has many features that make automation very simple. Do read more from their documentation page.