Linux Basics
Learn the basics of operating a Linux-based operating system (OS) and take your first steps in exploitation in a Linux environment!
Easy
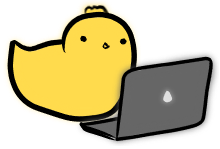
Linux_Basics::File_Manipulation
After knowing how to navigate through the file system, it is time to learn how to read from and write to files.
echo
The simplest way to write to a file is to use echo
. As the name suggests, echo
will echo any input it received as output on the terminal.
$ echo Hello World!
Hello World!
Now to write this to a file we can make use of the >
operator, which is a form of redirection operator in bash. This operator takes the output of the command on the left-hand side (LHS), and redirects it into the filename provided on the right-hand (RHS) side of the >
. Since the output of echo
is whatever arguments we provide to it, the following command can be used to write a simple string to a file.
$ echo Hello World! > hello_world
The command above would write the string Hello World!
into the file hello_world
in the current directory.
cat
To check the contents of hello_world
, we can use the cat
command.
$ cat hello_world
Hello World!
Here, we confirmed that Hello World!
is stored inside hello_world
.
> vs >>
Earlier, we tried using >
to redirect the output of echo
into a file. Try doing it again, but with a different input string to echo
.
$ echo New Input! > hello_world
$ cat hello_world
New Input!
Notice that Hello World!
is no longer in the file, and has been replaced by New Input!
.
This occurs because the >
will overwrite any existing contents of the target file.
If we want to apppend to the file instead of completely overwriting what was inside, we can use the >>
operator.
$ echo More Hellos! >> hello_world
$ cat hello_world
New Input!
More Hellos!
Quiz
To append a file without overwriting its contents, which redirection operator should we use?
nano
Using echo
with >
does not give the capability to modify file contents. Instead, we can use nano
, a small editor in the terminal. nano
is one of the easiest terminal-based editors to use. Here is a mini cheatsheet and demo.
# Usage:
nano <filename>
e.g.
$ nano hello_world
# Inside nano
+-------------+--------------------------------------------+
| Function | How to |
+-------------+--------------------------------------------|
| Navigation | Arrow Keys (Up, Down, Left, Right) |
| Edit Text | Type your text directly |
| Select Text | Shift + Arrow Keys (Up, Down, Left, Right) |
| Copy | (after selection) Alt + ^ [Shift+6] |
| Paste | Ctrl + U |
| Paste* | Ctrl + Shift + V |
| Undo | Alt + U |
| Redo | Alt + E |
| Exit | Ctrl + X |
+-------------+--------------------------------------------+
*paste from system clipboard, which is separate from nano's clipboard
less
Sometimes, a file may be very big, and it would not be a good idea to use cat
to print its contents. We don't want the terminal to be flooded with output. Take this password list with 200 lines for example. In this case, we can use the less
command, which allows us to scroll through the contents of a file.
As shown the demo above, we can scroll through the file with the mouse (if the terminal is configured to support this), or with the arrow keys. To exit, enter q
.
head/tail
Alternatively, we can use head
to print the first 10 lines of the file, or tail
for the last 10 lines.
$ head 2020-200_most_used_passwords.txt
123456
123456789
picture1
password
12345678
111111
123123
12345
1234567890
senha
$ tail 2020-200_most_used_passwords.txt
loveme
gabriel
alexander
cheese
passw0rd
142536
peanut
11223344
thomas
angel1
The number of lines printed can be customised using the -n
command-line flag.
$ head -n4 2020-200_most_used_passwords.txt
123456
123456789
picture1
password
$ tail -n4 2020-200_most_used_passwords.txt
peanut
11223344
thomas
angel1
grep
There is a very handy command grep
that filters lines containing a given pattern. The syntax is grep <PATTERN> <FILE>
.
$ grep password 2020-200_most_used_passwords.txt
password
password1
password123
Pipe
Earlier, we introduced the redirection operators (>
and >>
). The pipe operator (|
) behaves similarly, but instead of writing the output to a file, it sends the output of the command on the LHS as input to the command on the RHS.
The following examples are alternative ways to use head
, tail
and grep
.
Print the first 10 lines of 2020-200_most_used_passwords.txt
$ cat 2020-200_most_used_passwords.txt | head
123456
123456789
picture1
password
12345678
111111
123123
12345
1234567890
senha
Print the last 10 lines of 2020-200_most_used_passwords.txt
$ cat 2020-200_most_used_passwords.txt | tail
loveme
gabriel
alexander
cheese
passw0rd
142536
peanut
11223344
thomas
angel1
Print the lines of 2020-200_most_used_passwords.txt
that contain the substring password
$ cat 2020-200_most_used_passwords.txt | grep password
password
password1
password123
There is no difference between using pipes and using the commands directly (e.g. head file
). It is just a matter of preference.
When you've grasp this concept well you can achieve a lot just by chaining simple commands together with the pipe operator. Here is a command that gets the 6th to 10th passwords that contain 123
:
$ cat 2020-200_most_used_passwords.txt | grep 123 | head | tail -n5
1234567890
1234567
abc123
1234
123
Mini-Quiz
The quiz questions depend on the command line below.
Provide the appropriate command that goes into ...
to obtain the requested output.
$ cat passwords.txt | ...